ECS - Systems (Part 3)
- Richard Bradley
- Sep 2, 2019
- 2 min read
Updated: Sep 18, 2019
Systems
As both entities and components do not define behaviour, this burden falls to systems. These systems provide the logic for our game whilst also transforming the data of our components.
There are two available systems at time of writing.
ComponentSystem - Is a system that runs on Unity's main thread and therefore does not take advantage of multiple CPU cores. It runs operations on relevant entities and can not contain any instanced data, only methods.
JobComponentSystem - allows your game to take advantage of all your available CPU cores by creating a working thread per core therefore eliminating the cost of context switching. Unity also automatically takes care of race conditions so a lot of the hurdles of multi-threaded programming are taken care of for you
In this short tutorial I will discuss the commonalities between the aforementioned systems before moving onto the specifics of each them (in separate tutorials).
Life-cycle Event Functions
The ComponentSystemBase class, which all component systems inherit from, comes equipped with overridable life-cycle methods.
ECS executes these life-cycle methods on the main thread and in the following order:
OnCreate() - Invoked when a system is first created
OnStartRunning() - Invoked before the first OnUpdate() and when a the system resumes running.
OnUpdate() - If the system is Enabled and has work to do, then OnUpdate() is invoked every frame.
OnStopRunning() - Invoked before OnDestroy() and when a system has stopped updating due to no entities matching its queries.
OnDestroy() - Invoked when a system is destroyed.
The code snippet below shows a basic template for a ComponentSystem, including all available life-cycle methods.
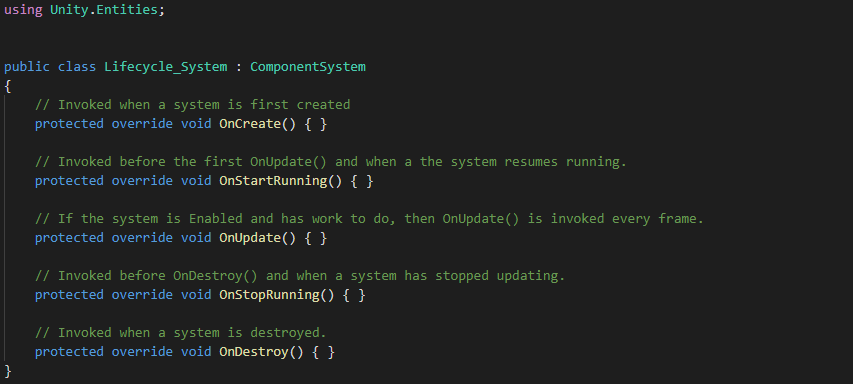
System Update Order
The order in which the ECS run-time executes systems is decided based upon which ComponentSystemGroup they belong to.
InitializationSystemGroup (updated at the end of the Initialization phase of the player loop)
SimulationSystemGroup (updated at the end of the Update phase of the player loop)
PresentationSystemGroup (updated at the end of the PreLateUpdate phase of the player loop)
To place a system in a group you can use the UpdateInGroup[typeof(T)] attribute (where T is the group type). If you do not place a system in a group explicitly then the ECS run-time will automatically place it in the default SimulationSystemGroup.
Below is an example of how to place a system in the PresentationSystemGroup.
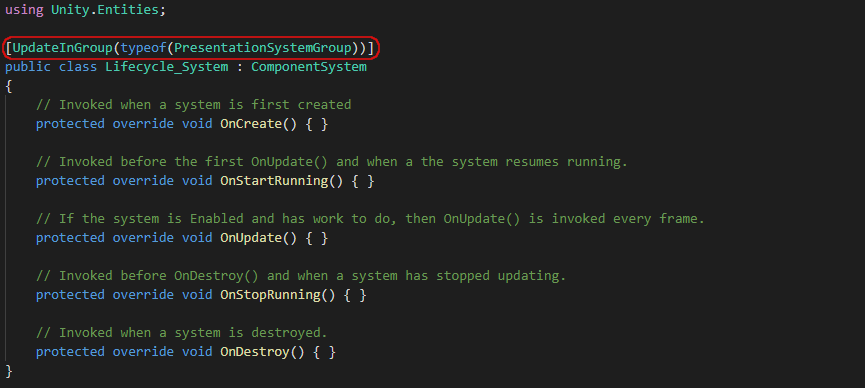
You can also control the system update order by using a UpdateAfter(typeof(T))] (where T is the system) attribute. Doing this tells Unity ECS to run the current system after another system has finished, as highlighted below.
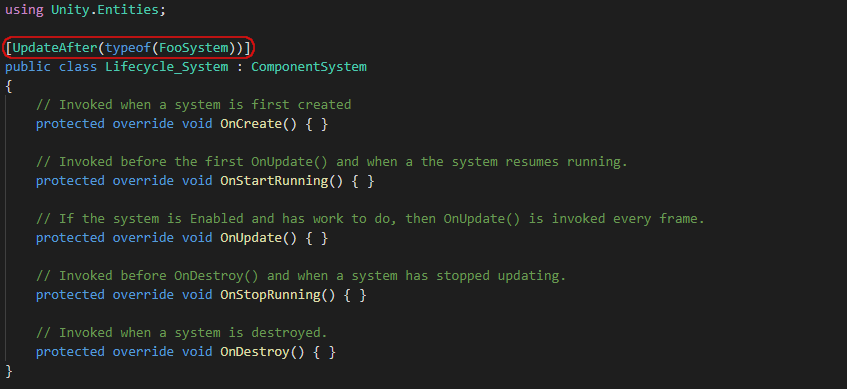
Likewise you can tell Unity ECS to execute the current system before another system is run by using the UpdateBefore(typeof(T))] as highlighted below.
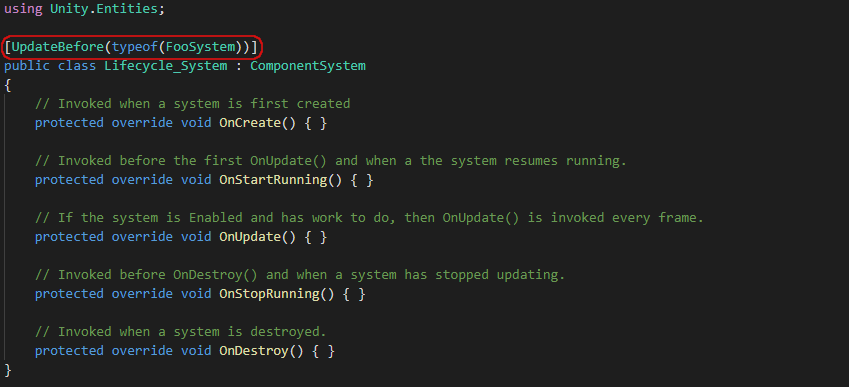
You can also combine both UpdateAfter(typeof(T))] and UpdateBefore(typeof(T))].
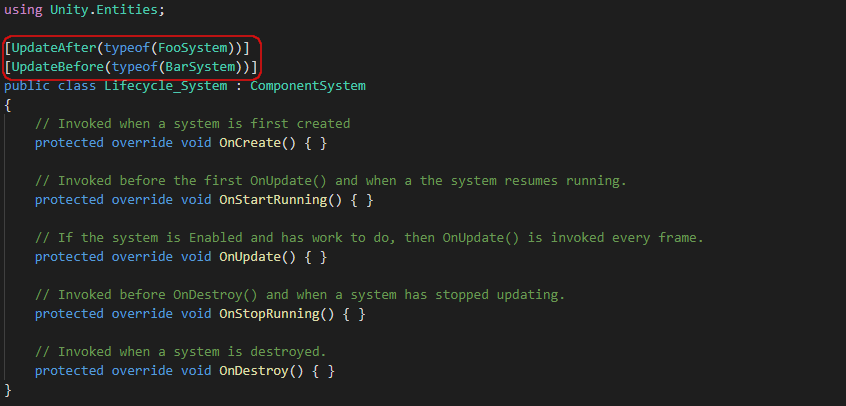
If you don't want Unity ECS to automatically run your systems at run-time then you can use a [DisableAutoCreation] attribute which will disable this functionality, allowing you to create and run the systems of your choosing via a Bootstrap script.
Highlighted below is an example of how to add the aforementioned attribute.
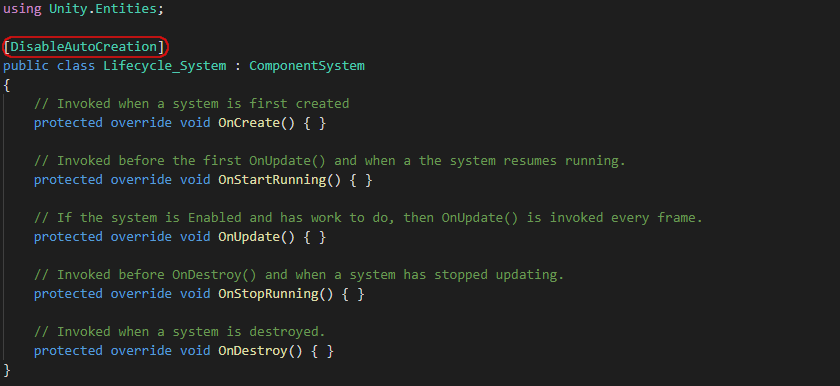
Now we know the basics of systems within Unity ECS, lets move onto the ComponentSystem specifics, which I will tackle in a separate tutorial (linked below)
Until next time.
Comments